How to Retrieve Data From Database in Java Using Eclipse
Hello guys, Welcome to another tutorial, and in this tutorial, we will learn how to connect MySQL database in Java using Eclipse with simple and easy steps. Most of the time, whenever we try to connect our program to the database, we face a lot of problems, and even then, we cannot connect successfully. But if you follow this tutorial properly, you will be able to connect your Java program to the MySQL database using Eclipse quite easily.
So Let's start our tutorial on how to connect MySQL database in Java using Eclipse.
How to Connect MySQL Database in Java Using Eclipse
Contents
- 1 How to Connect MySQL Database in Java Using Eclipse
- 1.1 Downloading and Installing Eclipse
- 1.2 Creating a Database and Table in MySQL
- 1.3 Creating a Java Project in Eclipse
- 1.4 Adding MySQL connector jar file in Eclipse
- 1.5 Connecting Java Program with MySQL Database
- 1.6 Inserting Data into The MySQL Database using Java
- 1.7 Updating Data in The MySQL Database using Java
- 1.8 How to Retrieve Data from MySQL Database in Java Using Eclipse
- 1.9 Deleting Data from the MySQL Database using Java
- 1.10 Conclusion
Downloading and Installing Eclipse
- The very First thing you need to do is to Download and Install Eclipse IDE on your system.
- Open your favorite browser and go to the official website of Eclipse www.eclipse.org .
- When you enter the official website of the eclipse, you will see a download button in the top right corner. Click on that.
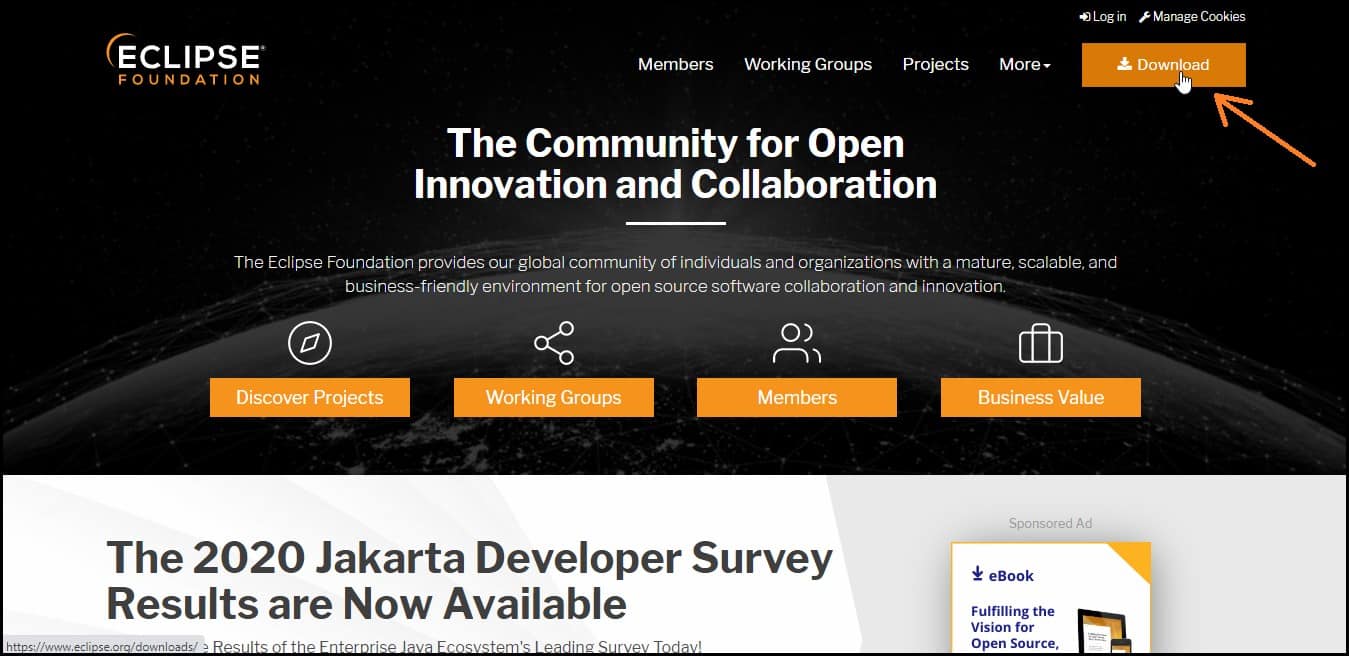
- After clicking on the Download Button, you will be redirected to another Web page. Click on the download button, as shown in the figure below.
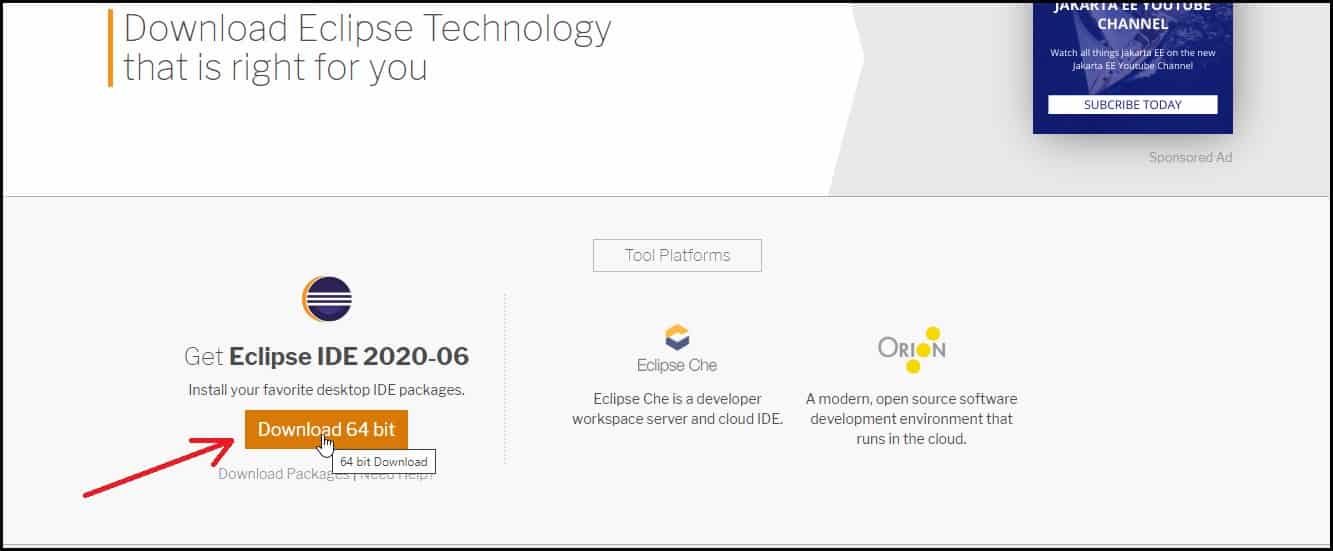
- Now again, click on the Download button to download the executable eclipse file.
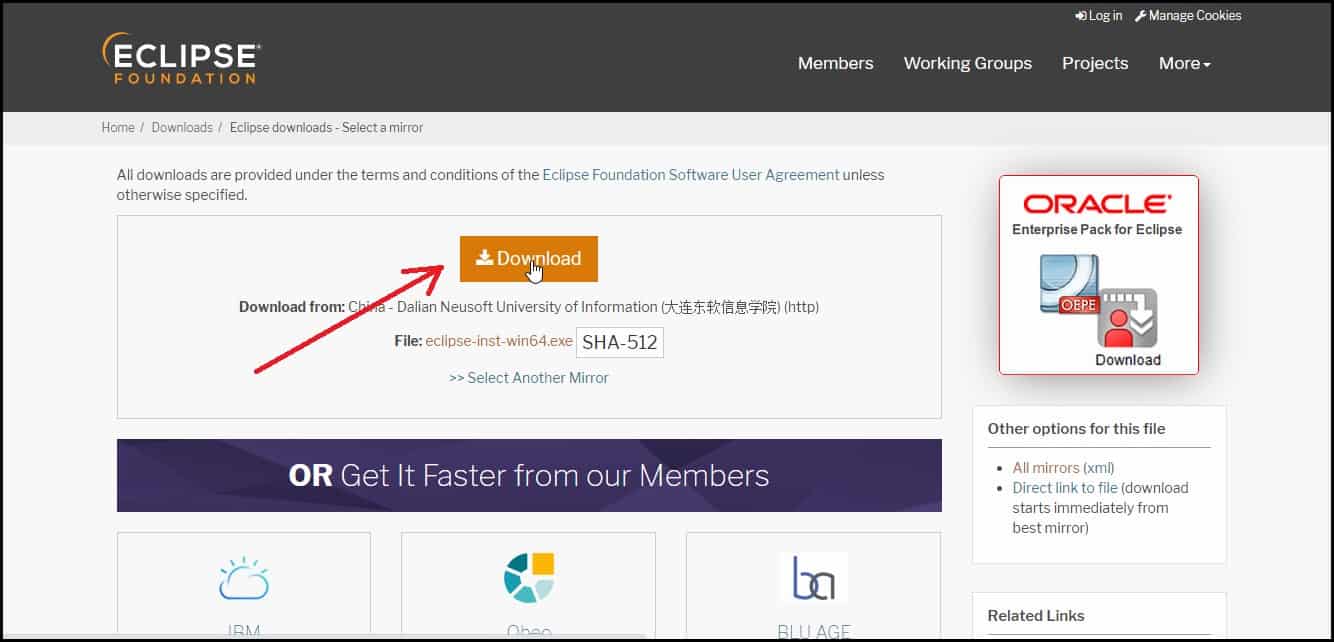
- After downloading the file, you need to install it in your system in the same way you install any application.
Creating a Database and Table in MySQL
- To create a database and table, you can either choose MySQL command-line client or MySQL workbench, which is nothing but a GUI version of the MySQL console where we can visually design, model, and generates databases.
- If you don't know how to install MySQL workbench in your system, then you can follow this guide, How to install MySQL Workbench .
- I will use MySQL command-line client in this tutorial, open MySQL command-line client, and enter your password so that you can connect the MySQL database.
- This is the same password you provided while installing the MySQL database in your system.
- Now create a database named "demodb" and a table named "student" inside it with columns
- ROLLNO
- USERNAME
- DEPT
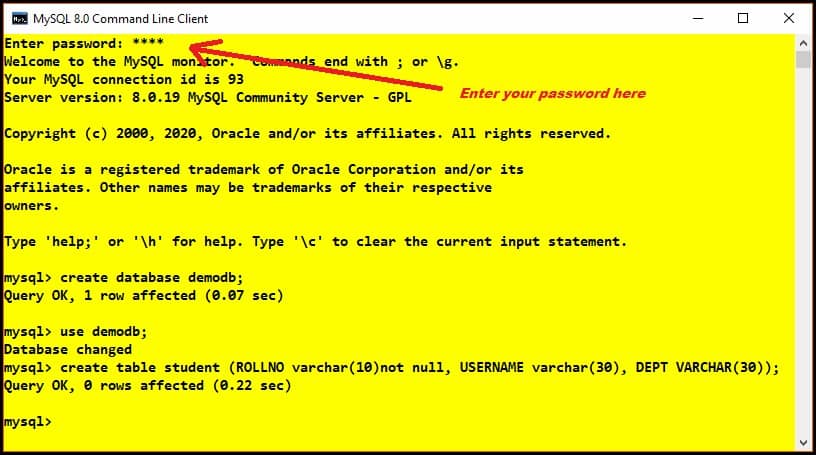
create database demodb ; use demodb ; create table student ( ROLLNO varchar ( 10 ) not null , USERNAME varchar ( 30 ) , DEPT VARCHAR ( 30 ) ) ; |
Creating a Java Project in Eclipse
- Open Eclipse IDE and click on the Java Project under the new section of File Menu ( File>>New>>Java Project ).
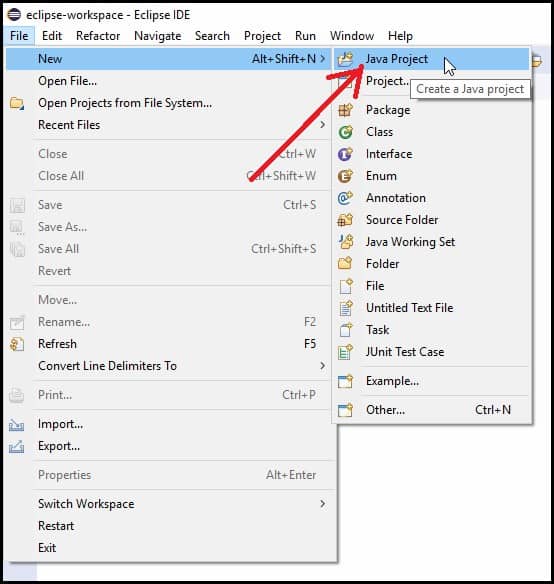
- Now give a name to your project (DbConnectin this example) and click on "Finish".
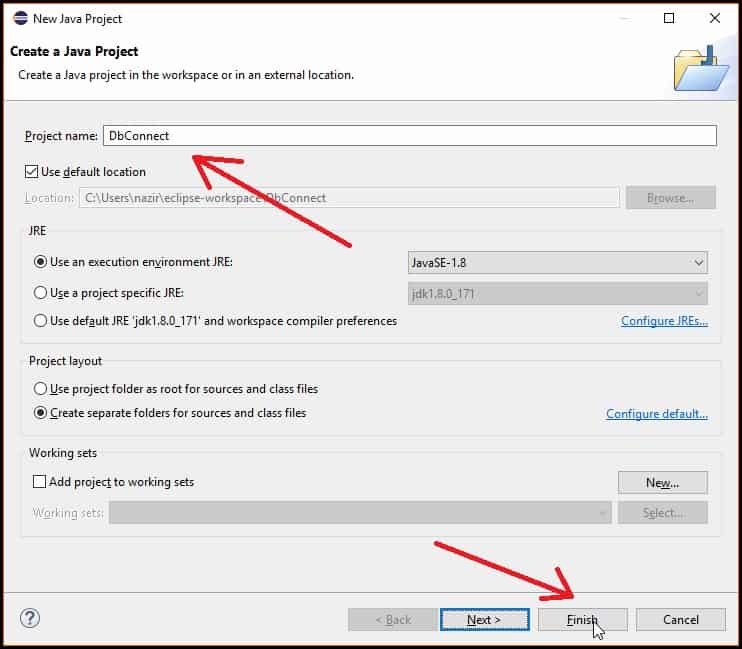
- Now right click on the project and create a new Java class ( New>>Class ).
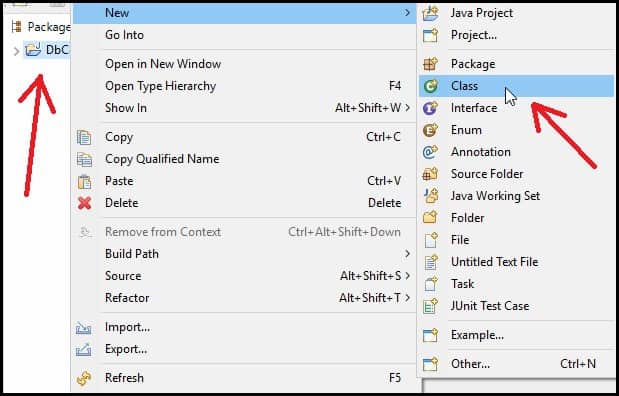
- Now give a name to your class(MyDbconnect in this Example), tick mark on the public static void main(String[] args), and then click on the Finish button as shown in the figure below.
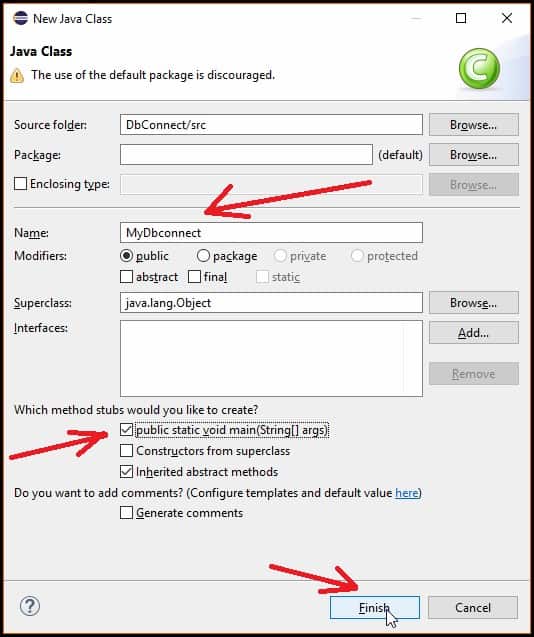
Adding MySQL connector jar file in Eclipse
- In order to connect your java program with MySQL database, you need to include MySQL JDBC driver which is a JAR file, namelymysql-connector-java-5.1.49-bin.jar. The version number in the Jar file can be different.
- You can download the latest version of MySQL connector from this link (MySQL Connector Java download) As shown in the figure below.
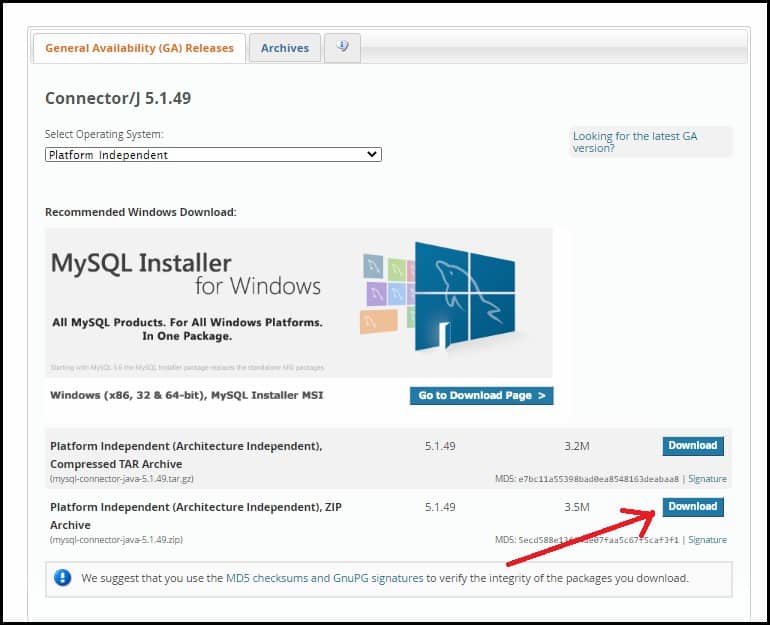
- Extract the zip archive and you will get the jar file.
- Next, you have to include this jar file into your program so that you will be able to connect your java program with MySQL database.
- Right-click on the project, go to the properties section then select Java Build Path and click on the Add External JARs button.
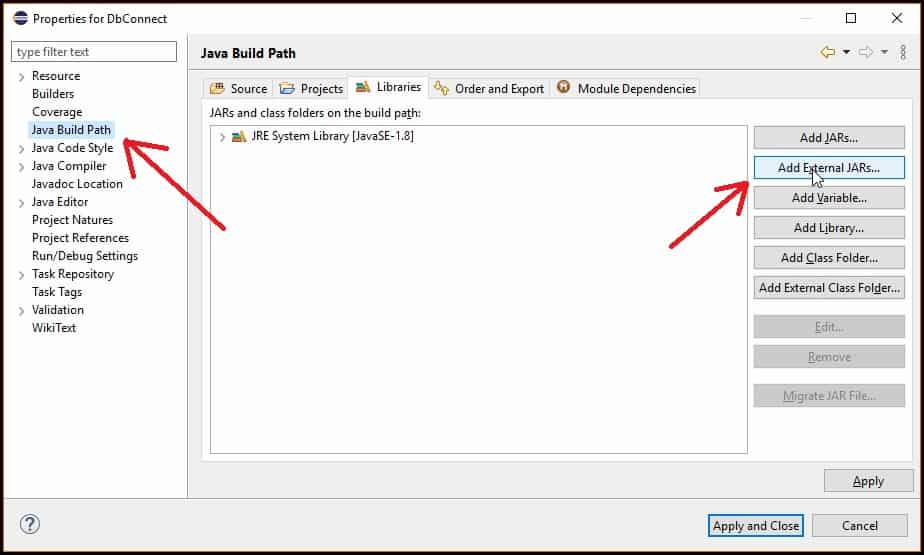
- After clicking on the button a pop-up window will appear where you have to select and open the jar file.
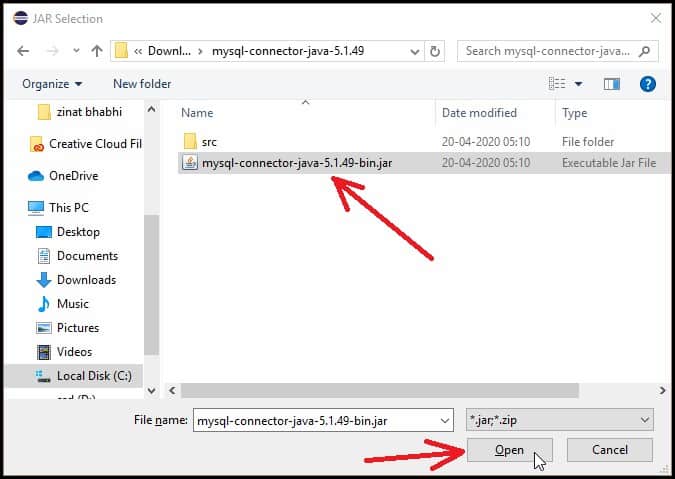
- You can see the added Jar file as shown in the figure below. Now click on theApply and Close button.
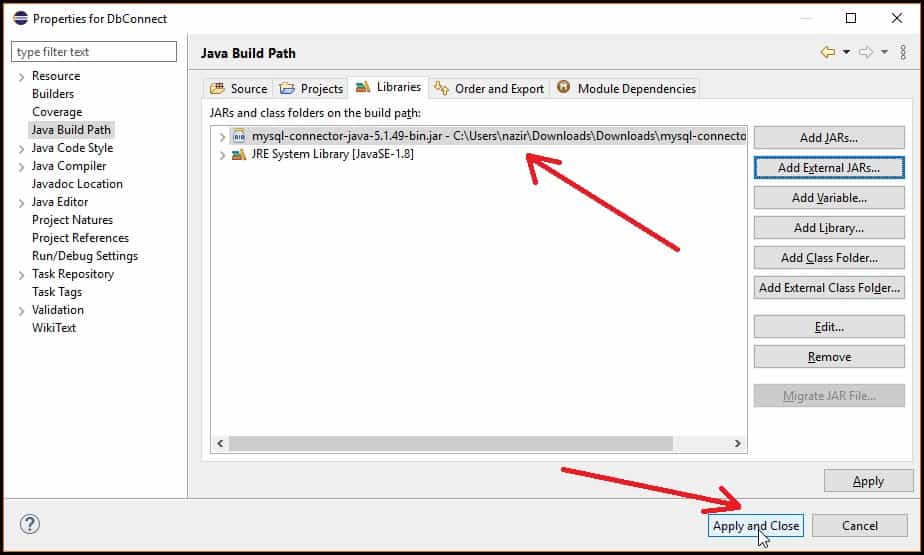
Connecting Java Program with MySQL Database
Since you have already finished adding jar file, now you are ready to connect your Java program with MySQL Database. Let's quickly take a look at the steps.
- Establish a connection using DriverManager.getConnection(String URL) and it returns a Connection reference.
- InString URL parameter you have to write like this jdbc:mysql://localhost:3306/demodb", "root", "root"where,
-
jdbc is the API.
- mysql is the database.
- localhost is the name of the server in which MySQL is running.
- 3306 is the port number.
- demodb is the database name. If your database name is different, then you have to replace this name with your database name.
- The first root is the username of the MySQL database. It is the default username for the MySQL database.
-
The second root is the password that you give while installing the MySQL database.
-
- SQL Exception might occur while connecting to the database, So you need to surround it with the try-catch block.
- Programming Example is given below.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 | import java . sql . * ; public class MyDbconnect { public static void main ( String [ ] args ) { try { Connection connection = DriverManager . getConnection ( "jdbc:mysql://localhost:3306/demodb" , "root" , "root" ) ; //Establishing connection System . out . println ( "Connected With the database successfully" ) ; } catch ( SQLException e ) { System . out . println ( "Error while connecting to the database" ) ; } } } |
Inserting Data into The MySQL Database using Java
As we saw right away how to connect MySQL database in Java Using Eclipse, now its time to insert some data into our table. If you are familiar with the Prepared Statement in Java and about SQL INSERT query , then it will be a lot of easier for you to add data to your table.
- Create a PreparedStatement object and use SQL INSERT query to add data to the table.
- Specify the values for each parameter through the PreparedStatement object.
- Execute the query through the PreparedStatement object.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 | import java . sql . * ; public class MyDbconnect { public static void main ( String [ ] args ) { try { Connection connection = DriverManager . getConnection ( "jdbc:mysql://localhost:3306/demodb" , "root" , "root" ) ; //Establishing connection System . out . println ( "Connected With the database successfully" ) ; //Crating PreparedStatement object PreparedStatement preparedStatement = connection . prepareStatement ( "insert into Student values(?,?,?)" ) ; //Setting values for Each Parameter preparedStatement . setString ( 1 , "1" ) ; preparedStatement . setString ( 2 , "Mehtab" ) ; preparedStatement . setNString ( 3 , "Computer" ) ; //Executing Query preparedStatement . executeUpdate ( ) ; System . out . println ( "data inserted successfully" ) ; } catch ( SQLException e ) { System . out . println ( "Error while connecting to the database" ) ; } } } |
- Now run your program.
- To check whether data is inserted in the table or not, open MySQL database and connect it by entering the password and write the following query.
use demodb ; select * from student ; |
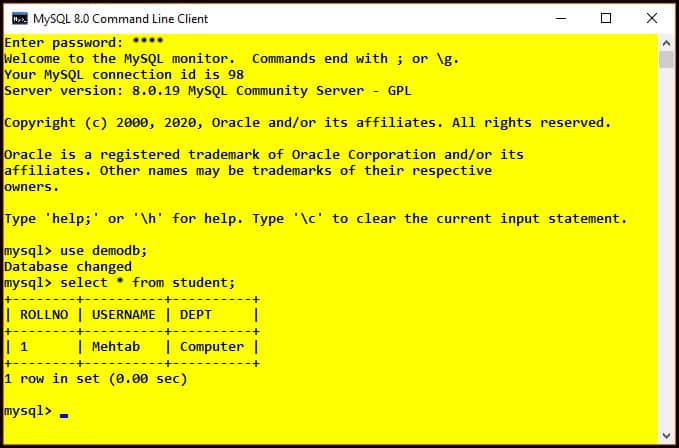
Updating Data in The MySQL Database using Java
- To update data in the table, you have to use SQL UPDATE query.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 | import java . sql . * ; public class MyDbconnect { public static void main ( String [ ] args ) { try { Connection connection = DriverManager . getConnection ( "jdbc:mysql://localhost:3306/demodb" , "root" , "root" ) ; //Establishing connection System . out . println ( "Connected With the database successfully" ) ; //Using SQL UPDATE query to update data in the table PreparedStatement preparedStatement = connection . prepareStatement ( "update Student set DEPT=? where ROLLNO=?" ) ; preparedStatement . setString ( 1 , "Physics" ) ; preparedStatement . setString ( 2 , "1" ) ; preparedStatement . executeUpdate ( ) ; System . out . println ( "data updated successfully" ) ; } catch ( SQLException e ) { System . out . println ( "Error while connecting to the database" ) ; } } } |
- Now run your program.
- To check whether data is inserted in the table or not, open MySQL database and connect it by entering the password and write the following query.
use demodb ; select * from student ; |
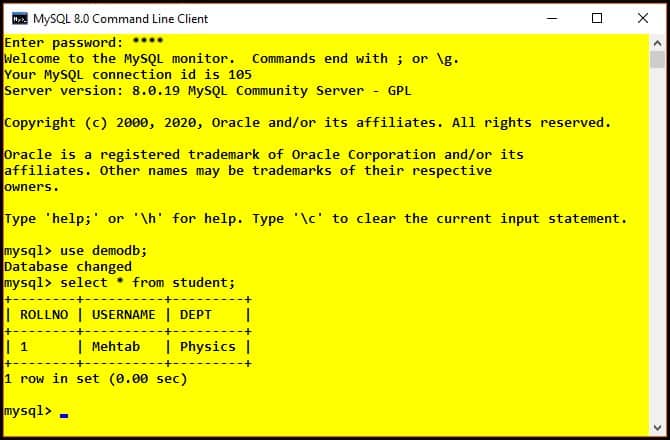
How to Retrieve Data from MySQL Database in Java Using Eclipse
- To retrieve data from the table, we will use SQL SELECT query.
- In order to get the results, we will use Java ResultSet object.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 | import java . sql . * ; public class MyDbconnect { public static void main ( String [ ] args ) { try { Connection connection = DriverManager . getConnection ( "jdbc:mysql://localhost:3306/demodb" , "root" , "root" ) ; //Establishing connection System . out . println ( "Connected With the database successfully" ) ; //Using SQL SELECT Query PreparedStatement preparedStatement = connection . prepareStatement ( "select * from Student" ) ; //Creating Java ResultSet object ResultSet resultSet = preparedStatement . executeQuery ( ) ; while ( resultSet . next ( ) ) { String rollNo = resultSet . getString ( "ROLLNO" ) ; String name = resultSet . getString ( "USERNAME" ) ; String dept = resultSet . getString ( "DEPT" ) ; //Printing Results System . out . println ( rollNo + " " + name + " " + dept ) ; } } catch ( SQLException e ) { System . out . println ( "Error while connecting to the database" ) ; } } } |
Deleting Data from the MySQL Database using Java
- To delete data from the table, we will use SQL DELETE query.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 | import java . sql . * ; public class MyDbconnect { public static void main ( String [ ] args ) { try { Connection connection = DriverManager . getConnection ( "jdbc:mysql://localhost:3306/demodb" , "root" , "root" ) ; //Establishing connection System . out . println ( "Connected With the database successfully" ) ; //Using SQL DELETE query PreparedStatement preparedStatement = connection . prepareStatement ( "delete from Student where Username=?" ) ; preparedStatement . setString ( 1 , "Mehtab" ) ; preparedStatement . executeUpdate ( ) ; System . out . println ( "Data deleted Successfully" ) ; } catch ( SQLException e ) { System . out . println ( "Error while connecting to the database" ) ; } } } |
Conclusion
Finally, We have concluded that connecting the MySQL database to Java is not as difficult as we think. All we need is to follow the steps correctly.
So, guys, I am wrapping up this tutorial "How to connect MySQL Database in Java Using Eclipse" and Feel free to drop us a comment if you find out something difficult to understand.
If you want to have a deep knowledge of Java Programming through Books, you can download the Beginners Java Programming Books in the PDF version for absolutely free by clicking on the below button.
People Are Also Reading…
- How to Play Mp3 File in Java Tutorial | Simple Steps
- Tic Tac Toe Java Code Against Computer With Source Code
- Calculator Program in Java Swing/JFrame with Source Code
- Registration Form in Java With Database Connectivity
- How to Create Login Form in Java Swing
- Text to Speech in Java
- How to Create Splash Screen in Java
- Java Button Click Event
- Menu Driven Program in Java
How to Retrieve Data From Database in Java Using Eclipse
Source: https://www.tutorialsfield.com/how-to-connect-mysql-database-in-java-using-eclipse/
0 Response to "How to Retrieve Data From Database in Java Using Eclipse"
Post a Comment